Consuming a AJAX-Enabled WCF Service in Client-Side AJAX ASP.NET C#
Step2) Add new item by selecting "Ajax-Enabled WCF Service" Name it as "ProfileWCFService"
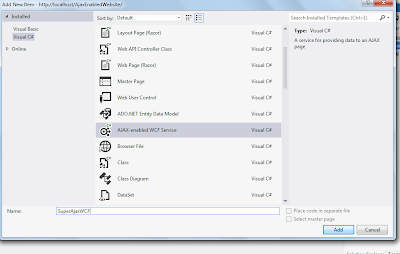
ProfileWCFService.cs
[ServiceContract(Namespace = "")]
[AspNetCompatibilityRequirements(RequirementsMode = AspNetCompatibilityRequirementsMode.Allowed)]
public class ProfileWCFService
{
// To use HTTP GET, add [WebGet] attribute. (Default ResponseFormat is WebMessageFormat.Json)
// To create an operation that returns XML,
// add [WebGet(ResponseFormat=WebMessageFormat.Xml)],
// and include the following line in the operation body:
// WebOperationContext.Current.OutgoingResponse.ContentType = "text/xml";
[OperationContract]
public void DoWork()
{
// Add your operation implementation here
return;
}
// Add more operations here and mark them with [OperationContract]
List<Profile> profiles = new List<Profile>();
[OperationContract]
public List<Profile> GetAllProfiles()
{
BuildProfile();
return profiles;
}
[OperationContract]
public Profile GetProfile(int ProfileID)
{
BuildProfile();
Profile profile = profiles.SingleOrDefault(p => p.ProfileID == ProfileID);
return profile;
}
private void BuildProfile()
{
if (profiles.Count == 0)
{
profiles.Add(new Profile { Age = 21, Country = "USA",
EmailID = "jhon@gmail.com", FirstName = "jhon",
LastName = "Peter", ProfileID = 1, ZIPCode = "94086-2422",
JoinedDate = new System.DateTime(1998, 1, 12) });
profiles.Add(new Profile { Age = 18, Country = "USA",
EmailID = "macy@yahoo.com", FirstName = "macy", LastName = "William",
ProfileID = 2, ZIPCode = "67897-2422",
JoinedDate = new System.DateTime(2000, 11, 1) });
profiles.Add(new Profile { Age = 28, Country = "UK", EmailID = "alex@gmail.com",
FirstName = "alex", LastName = "Samuel", ProfileID = 3, ZIPCode = "STHL 1ZZ",
JoinedDate = new System.DateTime(2002, 10, 9) });
profiles.Add(new Profile { Age = 35, Country = "UK", EmailID = "bearns@gmail.com",
FirstName = "beans", LastName = "Jessica", ProfileID = 4,
ZIPCode = "AA9A 9AA", JoinedDate = new System.DateTime(2004, 10, 12) });
profiles.Add(new Profile { Age = 36, Country = "INDIA", EmailID = "prasad@gmail.com", FirstName = "prasad", LastName = "kanuturi",
ProfileID = 5, ZIPCode = "110011",
JoinedDate = new System.DateTime(1990, 1, 2) });
}
}
[DataContract]
public class Profile
{
[DataMember]
public int ProfileID { get; set; }
[DataMember]
public String FirstName { get; set; }
[DataMember]
public String LastName { get; set; }
[DataMember]
public int Age { get; set; }
[DataMember]
public DateTime JoinedDate { get; set; }
[DataMember]
public String EmailID { get; set; }
[DataMember]
public String ZIPCode { get; set; }
[DataMember]
public String Country { get; set; }
}
}
Step 3) Add New item Web Form and name it as Default2.aspx

In the Default2.aspx
<Services>
<asp:ServiceReference Path="~/ProfileWCFService.svc" />
</Services>
</asp:ScriptManager>
<asp:Button ID="Button1" runat="server"
Text="Call Ajax Enabled WCF"
OnClientClick="javascript:calling_WCF_in_Javascript()" />
<asp:UpdatePanel ID="UpdatePanel1" runat="server">
<ContentTemplate>
<div style="color:green;font-size:2em;border-width:thick;border-color:red;"
id="wcf_ajax_response"
runat="server">
No Ajax Response
</div>
</ContentTemplate>
<Triggers>
<asp:AsyncPostBackTrigger ControlID="Button1" EventName="Click" />
</Triggers>
</asp:UpdatePanel>
Step 4) Add Javascript Code for Creating AJAX-WCF Service.
<head runat="server">
<title>AJAX-Enabled WCF Service Example</title>
<script type="text/javascript">
function calling_WCF_in_Javascript() {
try {
var proxy = new ProfileWCFService(); //Create a WCF Service in javascript
//note NameSpace is Empty.
//Calling WCF methods in javascript.
proxy.GetAllProfiles(onSuccess, OnFailed, null);
}
catch (error) {
alert(error.message);
}
}
function onSuccess(result) {
var str = "<table border='2'>";
if (Array.isArray(result)) {
for (var i = 0; i < result.length; i++) {
var dt = new Date(result[i].JoinedDate.toString());
str += "<tr>";
str += "<td>" + result[i].ProfileID + "</td>";
str += "<td>" + result[i].FirstName + "</td>";
str += "<td>" + result[i].LastName + "</td>";
str += "<td>" + result[i].Age + "</td>";
str += "<td>" + dt.getFullYear()+"/"+dt.getDate()+"/"+dt.getDay() + "</td>";
str += "<td>" + result[i].EmailID + "</td>";
str += "<td>" + result[i].ZIPCode + "</td>";
str += "<td>" + result[i].Country + "</td>";
dt.toString();
alert(dt.getDate());
}
str += "</tr>";
str += "</table>";
document.getElementById('wcf_ajax_response').innerHTML = str;
}
}
function OnFailed(result) {
alert(result);
}
</script>
</head>
Step 1) Create a Empty ASP.NET Website. Name it as AjaxEnableWebsite
Step2) Add new item by selecting "Ajax-Enabled WCF Service" Name it as "ProfileWCFService"
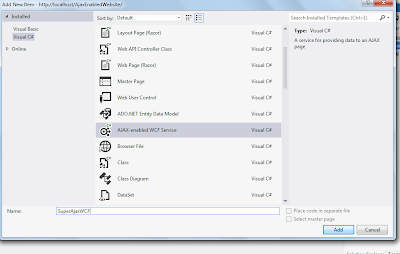
ProfileWCFService.cs
[ServiceContract(Namespace = "")]
[AspNetCompatibilityRequirements(RequirementsMode = AspNetCompatibilityRequirementsMode.Allowed)]
public class ProfileWCFService
{
// To use HTTP GET, add [WebGet] attribute. (Default ResponseFormat is WebMessageFormat.Json)
// To create an operation that returns XML,
// add [WebGet(ResponseFormat=WebMessageFormat.Xml)],
// and include the following line in the operation body:
// WebOperationContext.Current.OutgoingResponse.ContentType = "text/xml";
[OperationContract]
public void DoWork()
{
// Add your operation implementation here
return;
}
// Add more operations here and mark them with [OperationContract]
List<Profile> profiles = new List<Profile>();
[OperationContract]
public List<Profile> GetAllProfiles()
{
BuildProfile();
return profiles;
}
[OperationContract]
public Profile GetProfile(int ProfileID)
{
BuildProfile();
Profile profile = profiles.SingleOrDefault(p => p.ProfileID == ProfileID);
return profile;
}
private void BuildProfile()
{
if (profiles.Count == 0)
{
profiles.Add(new Profile { Age = 21, Country = "USA",
EmailID = "jhon@gmail.com", FirstName = "jhon",
LastName = "Peter", ProfileID = 1, ZIPCode = "94086-2422",
JoinedDate = new System.DateTime(1998, 1, 12) });
profiles.Add(new Profile { Age = 18, Country = "USA",
EmailID = "macy@yahoo.com", FirstName = "macy", LastName = "William",
ProfileID = 2, ZIPCode = "67897-2422",
JoinedDate = new System.DateTime(2000, 11, 1) });
profiles.Add(new Profile { Age = 28, Country = "UK", EmailID = "alex@gmail.com",
FirstName = "alex", LastName = "Samuel", ProfileID = 3, ZIPCode = "STHL 1ZZ",
JoinedDate = new System.DateTime(2002, 10, 9) });
profiles.Add(new Profile { Age = 35, Country = "UK", EmailID = "bearns@gmail.com",
FirstName = "beans", LastName = "Jessica", ProfileID = 4,
ZIPCode = "AA9A 9AA", JoinedDate = new System.DateTime(2004, 10, 12) });
profiles.Add(new Profile { Age = 36, Country = "INDIA", EmailID = "prasad@gmail.com", FirstName = "prasad", LastName = "kanuturi",
ProfileID = 5, ZIPCode = "110011",
JoinedDate = new System.DateTime(1990, 1, 2) });
}
}
[DataContract]
public class Profile
{
[DataMember]
public int ProfileID { get; set; }
[DataMember]
public String FirstName { get; set; }
[DataMember]
public String LastName { get; set; }
[DataMember]
public int Age { get; set; }
[DataMember]
public DateTime JoinedDate { get; set; }
[DataMember]
public String EmailID { get; set; }
[DataMember]
public String ZIPCode { get; set; }
[DataMember]
public String Country { get; set; }
}
}
Step 3) Add New item Web Form and name it as Default2.aspx

In the Default2.aspx
- Add ScriptManager
- Add Button Server Control
- UpdatePanel
<Services>
<asp:ServiceReference Path="~/ProfileWCFService.svc" />
</Services>
</asp:ScriptManager>
<asp:Button ID="Button1" runat="server"
Text="Call Ajax Enabled WCF"
OnClientClick="javascript:calling_WCF_in_Javascript()" />
<asp:UpdatePanel ID="UpdatePanel1" runat="server">
<ContentTemplate>
<div style="color:green;font-size:2em;border-width:thick;border-color:red;"
id="wcf_ajax_response"
runat="server">
No Ajax Response
</div>
</ContentTemplate>
<Triggers>
<asp:AsyncPostBackTrigger ControlID="Button1" EventName="Click" />
</Triggers>
</asp:UpdatePanel>
Step 4) Add Javascript Code for Creating AJAX-WCF Service.
<head runat="server">
<title>AJAX-Enabled WCF Service Example</title>
<script type="text/javascript">
function calling_WCF_in_Javascript() {
try {
var proxy = new ProfileWCFService(); //Create a WCF Service in javascript
//note NameSpace is Empty.
//Calling WCF methods in javascript.
proxy.GetAllProfiles(onSuccess, OnFailed, null);
}
catch (error) {
alert(error.message);
}
}
function onSuccess(result) {
var str = "<table border='2'>";
if (Array.isArray(result)) {
for (var i = 0; i < result.length; i++) {
var dt = new Date(result[i].JoinedDate.toString());
str += "<tr>";
str += "<td>" + result[i].ProfileID + "</td>";
str += "<td>" + result[i].FirstName + "</td>";
str += "<td>" + result[i].LastName + "</td>";
str += "<td>" + result[i].Age + "</td>";
str += "<td>" + dt.getFullYear()+"/"+dt.getDate()+"/"+dt.getDay() + "</td>";
str += "<td>" + result[i].EmailID + "</td>";
str += "<td>" + result[i].ZIPCode + "</td>";
str += "<td>" + result[i].Country + "</td>";
dt.toString();
alert(dt.getDate());
}
str += "</tr>";
str += "</table>";
document.getElementById('wcf_ajax_response').innerHTML = str;
}
}
function OnFailed(result) {
alert(result);
}
</script>
</head>
Step 5) Run the Application
Complete Source Code
ProfileWCFService.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Runtime.Serialization;
using System.ServiceModel;
using System.ServiceModel.Activation;
using System.ServiceModel.Web;
using System.Text;
[ServiceContract(Namespace = "")]
[AspNetCompatibilityRequirements(RequirementsMode = AspNetCompatibilityRequirementsMode.Allowed)]
public class ProfileWCFService
{
// To use HTTP GET, add [WebGet] attribute. (Default ResponseFormat is WebMessageFormat.Json)
// To create an operation that returns XML,
// add [WebGet(ResponseFormat=WebMessageFormat.Xml)],
// and include the following line in the operation body:
// WebOperationContext.Current.OutgoingResponse.ContentType = "text/xml";
[OperationContract]
public void DoWork()
{
// Add your operation implementation here
return;
}
// Add more operations here and mark them with [OperationContract]
List<Profile> profiles = new List<Profile>();
[OperationContract]
public List<Profile> GetAllProfiles()
{
BuildProfile();
return profiles;
}
[OperationContract]
public Profile GetProfile(int ProfileID)
{
BuildProfile();
Profile profile = profiles.SingleOrDefault(p => p.ProfileID == ProfileID);
return profile;
}
private void BuildProfile()
{
if (profiles.Count == 0)
{
profiles.Add(new Profile { Age = 21, Country = "USA", EmailID = "jhon@gmail.com", FirstName = "jhon", LastName = "Peter", ProfileID = 1, ZIPCode = "94086-2422", JoinedDate = new System.DateTime(1998, 1, 12) });
profiles.Add(new Profile { Age = 18, Country = "USA", EmailID = "macy@yahoo.com", FirstName = "macy", LastName = "William", ProfileID = 2, ZIPCode = "67897-2422", JoinedDate = new System.DateTime(2000, 11, 1) });
profiles.Add(new Profile { Age = 28, Country = "UK", EmailID = "alex@gmail.com", FirstName = "alex", LastName = "Samuel", ProfileID = 3, ZIPCode = "STHL 1ZZ", JoinedDate = new System.DateTime(2002, 10, 9) });
profiles.Add(new Profile { Age = 35, Country = "UK", EmailID = "bearns@gmail.com", FirstName = "beans", LastName = "Jessica", ProfileID = 4, ZIPCode = "AA9A 9AA", JoinedDate = new System.DateTime(2004, 10, 12) });
profiles.Add(new Profile { Age = 36, Country = "INDIA", EmailID = "prasad@gmail.com", FirstName = "prasad", LastName = "kanuturi", ProfileID = 5, ZIPCode = "110011", JoinedDate = new System.DateTime(1990, 1, 2) });
}
}
[DataContract]
public class Profile
{
[DataMember]
public int ProfileID { get; set; }
[DataMember]
public String FirstName { get; set; }
[DataMember]
public String LastName { get; set; }
[DataMember]
public int Age { get; set; }
[DataMember]
public DateTime JoinedDate { get; set; }
[DataMember]
public String EmailID { get; set; }
[DataMember]
public String ZIPCode { get; set; }
[DataMember]
public String Country { get; set; }
}
}
using System.Collections.Generic;
using System.Linq;
using System.Runtime.Serialization;
using System.ServiceModel;
using System.ServiceModel.Activation;
using System.ServiceModel.Web;
using System.Text;
[ServiceContract(Namespace = "")]
[AspNetCompatibilityRequirements(RequirementsMode = AspNetCompatibilityRequirementsMode.Allowed)]
public class ProfileWCFService
{
// To use HTTP GET, add [WebGet] attribute. (Default ResponseFormat is WebMessageFormat.Json)
// To create an operation that returns XML,
// add [WebGet(ResponseFormat=WebMessageFormat.Xml)],
// and include the following line in the operation body:
// WebOperationContext.Current.OutgoingResponse.ContentType = "text/xml";
[OperationContract]
public void DoWork()
{
// Add your operation implementation here
return;
}
// Add more operations here and mark them with [OperationContract]
List<Profile> profiles = new List<Profile>();
[OperationContract]
public List<Profile> GetAllProfiles()
{
BuildProfile();
return profiles;
}
[OperationContract]
public Profile GetProfile(int ProfileID)
{
BuildProfile();
Profile profile = profiles.SingleOrDefault(p => p.ProfileID == ProfileID);
return profile;
}
private void BuildProfile()
{
if (profiles.Count == 0)
{
profiles.Add(new Profile { Age = 21, Country = "USA", EmailID = "jhon@gmail.com", FirstName = "jhon", LastName = "Peter", ProfileID = 1, ZIPCode = "94086-2422", JoinedDate = new System.DateTime(1998, 1, 12) });
profiles.Add(new Profile { Age = 18, Country = "USA", EmailID = "macy@yahoo.com", FirstName = "macy", LastName = "William", ProfileID = 2, ZIPCode = "67897-2422", JoinedDate = new System.DateTime(2000, 11, 1) });
profiles.Add(new Profile { Age = 28, Country = "UK", EmailID = "alex@gmail.com", FirstName = "alex", LastName = "Samuel", ProfileID = 3, ZIPCode = "STHL 1ZZ", JoinedDate = new System.DateTime(2002, 10, 9) });
profiles.Add(new Profile { Age = 35, Country = "UK", EmailID = "bearns@gmail.com", FirstName = "beans", LastName = "Jessica", ProfileID = 4, ZIPCode = "AA9A 9AA", JoinedDate = new System.DateTime(2004, 10, 12) });
profiles.Add(new Profile { Age = 36, Country = "INDIA", EmailID = "prasad@gmail.com", FirstName = "prasad", LastName = "kanuturi", ProfileID = 5, ZIPCode = "110011", JoinedDate = new System.DateTime(1990, 1, 2) });
}
}
[DataContract]
public class Profile
{
[DataMember]
public int ProfileID { get; set; }
[DataMember]
public String FirstName { get; set; }
[DataMember]
public String LastName { get; set; }
[DataMember]
public int Age { get; set; }
[DataMember]
public DateTime JoinedDate { get; set; }
[DataMember]
public String EmailID { get; set; }
[DataMember]
public String ZIPCode { get; set; }
[DataMember]
public String Country { get; set; }
}
}
ProfileWCFService.svc
<%@ ServiceHost Language="C#" Debug="true" Service="ProfileWCFService" CodeBehind="~/App_Code/ProfileWCFService.cs" %>
Default2.aspx
<%@ Page Language="C#" AutoEventWireup="true" CodeFile="Default2.aspx.cs" Inherits="Default2" %>
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<title>AJAX-Enabled WCF Service Example</title>
<style type="text/css">
.buttonStyle
{
color:white;
background-color:darkblue;
border-radius:10px;
}
</style>
<script type="text/javascript">
function calling_WCF_in_Javascript() {
try {
var proxy = new ProfileWCFService(); //Create a WCF Service in javascript
//note NameSpace is Empty.
//Calling WCF methods in javascript.
proxy.GetAllProfiles(onSuccess, OnFailed, null);
}
catch (error) {
alert(error.message);
}
}
function onSuccess(result) {
var str = "<table border='2'>";
if (Array.isArray(result)) {
for (var i = 0; i < result.length; i++) {
var dt = new Date(result[i].JoinedDate.toString());
str += "<tr>";
str += "<td>" + result[i].ProfileID + "</td>";
str += "<td>" + result[i].FirstName + "</td>";
str += "<td>" + result[i].LastName + "</td>";
str += "<td>" + result[i].Age + "</td>";
str += "<td>" + dt.getFullYear()+"/"+dt.getDate()+"/"+dt.getDay() + "</td>";
str += "<td>" + result[i].EmailID + "</td>";
str += "<td>" + result[i].ZIPCode + "</td>";
str += "<td>" + result[i].Country + "</td>";
dt.toString();
alert(dt.getDate());
}
str += "</tr>";
str += "</table>";
document.getElementById('wcf_ajax_response').innerHTML = str;
}
}
function OnFailed(result) {
alert(result);
}
</script>
</head>
<body>
<form id="form1" runat="server">
<div>
<asp:ScriptManager ID="ScriptManager1" runat="server" EnablePartialRendering="true">
<Services>
<asp:ServiceReference Path="~/ProfileWCFService.svc" />
</Services>
</asp:ScriptManager>
<br /><br />
<asp:Button CssClass="buttonStyle" ID="Button1" runat="server" Text="Call Ajax Enabled WCF Service" title="Call Ajax Enabled WCF Service returning complex object"
OnClientClick="javascript:calling_WCF_in_Javascript()" />
<asp:UpdatePanel ID="UpdatePanel1" runat="server">
<ContentTemplate>
<div style="color:green;font-size:2em;border-width:thick;border-color:red;"
id="wcf_ajax_response"
runat="server">
No Ajax Response
</div>
</ContentTemplate>
<Triggers>
<asp:AsyncPostBackTrigger ControlID="Button1" EventName="Click" />
</Triggers>
</asp:UpdatePanel>
</div>
</form>
</body>
</html>
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<title>AJAX-Enabled WCF Service Example</title>
<style type="text/css">
.buttonStyle
{
color:white;
background-color:darkblue;
border-radius:10px;
}
</style>
<script type="text/javascript">
function calling_WCF_in_Javascript() {
try {
var proxy = new ProfileWCFService(); //Create a WCF Service in javascript
//note NameSpace is Empty.
//Calling WCF methods in javascript.
proxy.GetAllProfiles(onSuccess, OnFailed, null);
}
catch (error) {
alert(error.message);
}
}
function onSuccess(result) {
var str = "<table border='2'>";
if (Array.isArray(result)) {
for (var i = 0; i < result.length; i++) {
var dt = new Date(result[i].JoinedDate.toString());
str += "<tr>";
str += "<td>" + result[i].ProfileID + "</td>";
str += "<td>" + result[i].FirstName + "</td>";
str += "<td>" + result[i].LastName + "</td>";
str += "<td>" + result[i].Age + "</td>";
str += "<td>" + dt.getFullYear()+"/"+dt.getDate()+"/"+dt.getDay() + "</td>";
str += "<td>" + result[i].EmailID + "</td>";
str += "<td>" + result[i].ZIPCode + "</td>";
str += "<td>" + result[i].Country + "</td>";
dt.toString();
alert(dt.getDate());
}
str += "</tr>";
str += "</table>";
document.getElementById('wcf_ajax_response').innerHTML = str;
}
}
function OnFailed(result) {
alert(result);
}
</script>
</head>
<body>
<form id="form1" runat="server">
<div>
<asp:ScriptManager ID="ScriptManager1" runat="server" EnablePartialRendering="true">
<Services>
<asp:ServiceReference Path="~/ProfileWCFService.svc" />
</Services>
</asp:ScriptManager>
<br /><br />
<asp:Button CssClass="buttonStyle" ID="Button1" runat="server" Text="Call Ajax Enabled WCF Service" title="Call Ajax Enabled WCF Service returning complex object"
OnClientClick="javascript:calling_WCF_in_Javascript()" />
<asp:UpdatePanel ID="UpdatePanel1" runat="server">
<ContentTemplate>
<div style="color:green;font-size:2em;border-width:thick;border-color:red;"
id="wcf_ajax_response"
runat="server">
No Ajax Response
</div>
</ContentTemplate>
<Triggers>
<asp:AsyncPostBackTrigger ControlID="Button1" EventName="Click" />
</Triggers>
</asp:UpdatePanel>
</div>
</form>
</body>
</html>
No comments:
Post a Comment